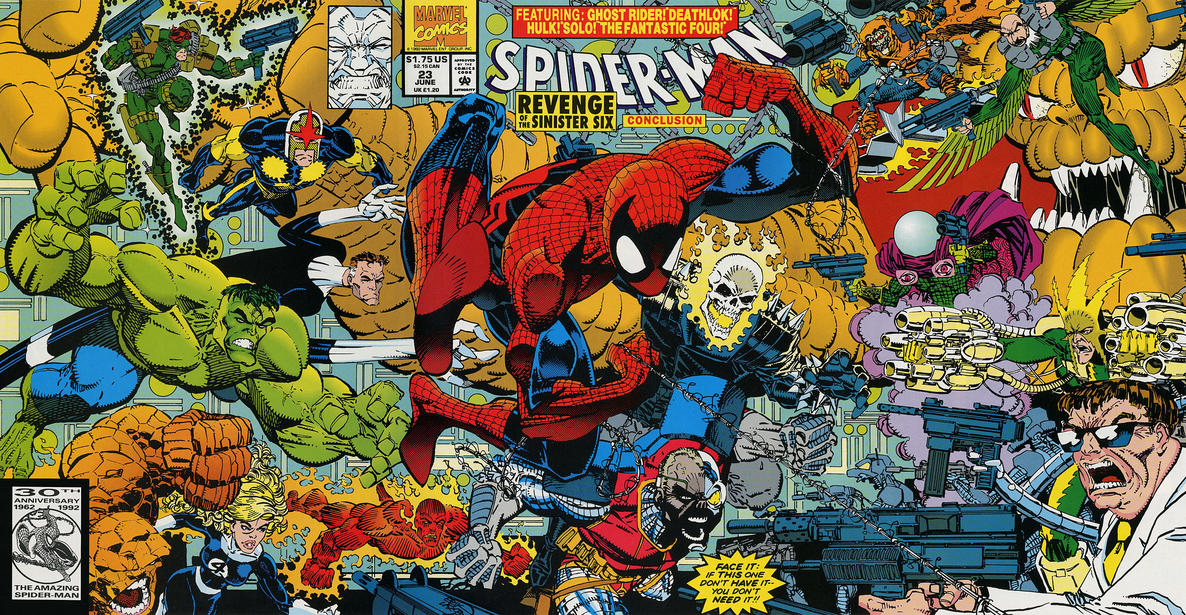
This post is mainly for my reference.. Well, to be honest all the posts are for my reference. Anyhoo, here is a few scripts which are concerned with created web servers or clients, or both!
I’ve tried to cover all the languages I’ve ever used and only a very minimal example for each.
I’m not sure whats going on with Java, it seems to be getting sucked into an enterprise Segmentum Obscurus..
Microsoft Dot Net 5+ (Client)
using System.Net;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Text.Json;
Action clientSend=()=>{
var socketsHandler=new SocketsHttpHandler(){
PooledConnectionLifetime=TimeSpan.FromMinutes(1)
};
var httpClient=new HttpClient(){
BaseAddress=new Uri("Http://127.0.0.1:8080")
};
using StringContent content=new(
JsonSerializer.Serialize(new{
userId=77,
title="title"+Guid.NewGuid().ToString()
})
);
var msg=new HttpRequestMessage(HttpMethod.Post,"companies");
msg.Content=content;
msg.Headers.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
msg.Content.Headers.ContentType=new MediaTypeHeaderValue("application/json");
Console.Write(".");
var resp=httpClient.Send(msg);
};
for(var i=0;i<100;i++){
Thread.Sleep(100);
Parallel.For(0, 1000, i =>
{
clientSend();
});
}
Microsoft .Net 5+ (Server)
using System.Net;
using System.Diagnostics;
namespace httpserver1;
public static class Program{
static HttpListener _listener=new HttpListener();
public static void ListenerCallback(IAsyncResult ar){
var context= _listener.EndGetContext(ar);
context.Response.StatusCode=200;
context.Response.StatusDescription="OK";
Console.Write("R");
context.Response.Close();
}
public static void ProcessRequests(){
var result = _listener.BeginGetContext(ListenerCallback, _listener);
var startNew = Stopwatch.StartNew();
result.AsyncWaitHandle.WaitOne();
startNew.Stop();
}
public static void Main(){
_listener=new HttpListener();
_listener.Prefixes.Add("http://*:8080/");
_listener.Start();
while(true){
ProcessRequests();
}
}
}
Microsoft – Webclient
using WebClient client = new WebClient();
using Stream d= client.OpenRead("http://localhost:8080");
using StreamReader r= new StreamReader(d);
string s = r.ReadToEnd();
Console.WriteLine(s);
NodeJS – Express (Server)
const exp = require('express')
const app = exp()
const port = 8080
app.get('/', (req, res) => {
res.send('Wow, just. Wow.')
})
app.listen(port, () => {
console.log(`Anything there on port ${port}`)
})
NodeJS – (http Client)
const http = require('http');
http.get('http://localhost:8080',(res)=>{
console.log(res);});
NodeJS – Axios (http Client)
const axios = require('axios');
http.get('http://localhost:8080')
.then(resp=>{
console.log(resp);})
.catch(err=>{console.log('error:'+resp);});
NodeJS – Got (http Client)
const got=require('got');
got.get('http://localhost:8080',{responseType:'json'})
.then(resp=>{
console.log('status',resp.statusCode);
})
.catch(err=>{console.log(err);});
Rails – httpClient
clnt = HTTPClient.new
clnt.get_content('http://localhost:8080/') do |chunk|
puts chunk
end
PHP – Guzzle
$cl= new GuzzleHttp\Client();
$resp = $client->request('GET', 'http://localhost:8080');
echo $resp->getBody();
Python
import http.client
conn= http.client.HTTPConnection("localhost:8080")
conn.request("GET", "/")
resp= conn.getresponse()
print("Status: {}".format(resp.status))
connection.close()
Perl
use HTTP::Tiny;
my $resp= HTTP::Tiny->new->get('http://localhost:8080/');
die "Failed!\n" unless $resp->{success};
print "$resp->{status}\n";
Java – Http request Builder
HttpResponse<String> resp= HttpClient.newBuilder()
.uri(new URI("http://localhost:8080"))
.build()
.send(request);
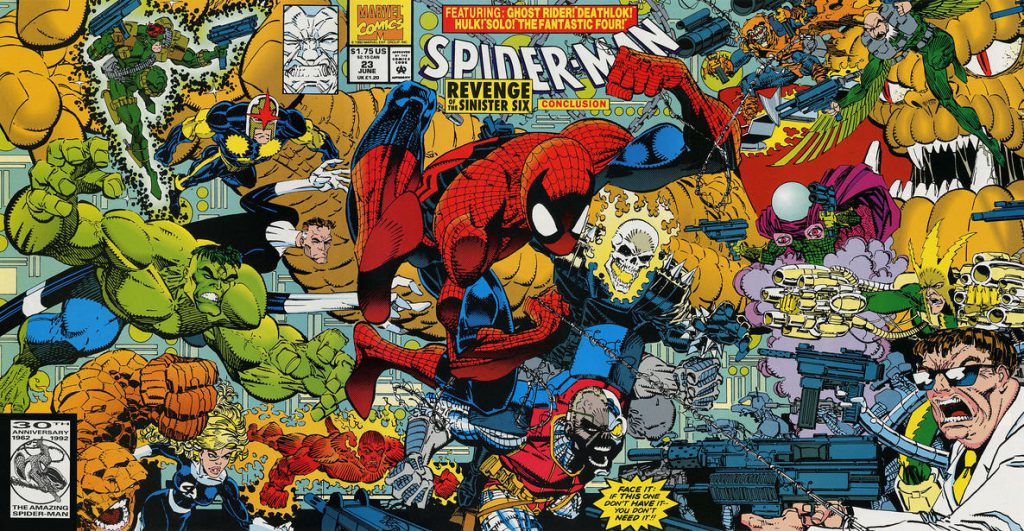